bytes
A sequence of bytes.
This is conceptually similar to an array of integers between 0
and 255
, but represented much more efficiently.
You can convert
- a string or an array of integers to bytes with the
bytes
constructor - bytes to a string with the
str
constructor - bytes to an array of integers with the
array
constructor
When reading data from a file, you can decide whether to load it as a string or as raw bytes.
#bytes((123, 160, 22, 0)) \
#bytes("Hello 😃")
#let data = read(
"rhino.png",
encoding: none,
)
// Magic bytes.
#array(data.slice(0, 4)) \
#str(data.slice(1, 4))
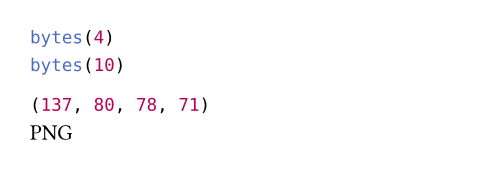
构造函数
如果类型具有构造函数,可以像函数一样调用它来创建一个该类型的值。
Converts a value to bytes.
- Strings are encoded in UTF-8.
- Arrays of integers between
0
and255
are converted directly. The dedicated byte representation is much more efficient than the array representation and thus typically used for large byte buffers (e.g. image data).
#bytes("Hello 😃") \
#bytes((123, 160, 22, 0))
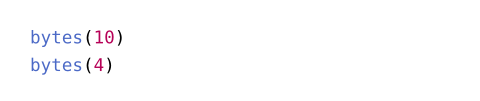
value
The value that should be converted to bytes.
定义
函数和类型可以有与其关联的定义 (成员或方法)。可以使用 "." 操作符来访问调用它们。
len
The length in bytes.
at
Returns the byte at the specified index. Returns the default value if the index is out of bounds or fails with an error if no default value was specified.
index
The index at which to retrieve the byte.
default
A default value to return if the index is out of bounds.
slice
Extracts a subslice of the bytes. Fails with an error if the start or index is out of bounds.
start
The start index (inclusive).
end
The end index (exclusive). If omitted, the whole slice until the end is extracted.
默认:none
count
The number of items to extract. This is equivalent to passing
start + count
as the end
position. Mutually exclusive with
end
.