array
A sequence of values.
You can construct an array by enclosing a comma-separated sequence of values in parentheses. The values do not have to be of the same type.
You can access and update array items with the .at()
method. Indices are
zero-based and negative indices wrap around to the end of the array. You can
iterate over an array using a for loop. Arrays can be
added together with the +
operator, joined together
and multiplied with integers.
Note: An array of length one needs a trailing comma, as in (1,)
.
This is to disambiguate from a simple parenthesized expressions like (1 + 2) * 3
. An empty array is written as ()
.
Example
#let values = (1, 7, 4, -3, 2)
#values.at(0) \
#(values.at(0) = 3)
#values.at(-1) \
#values.find(calc.even) \
#values.filter(calc.odd) \
#values.map(calc.abs) \
#values.rev() \
#(1, (2, 3)).flatten() \
#(("A", "B", "C")
.join(", ", last: " and "))
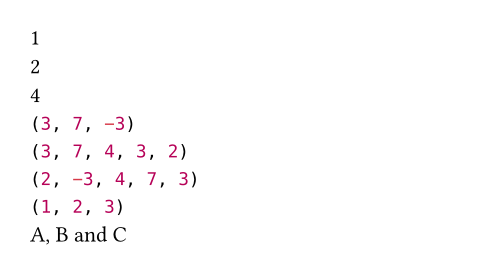
构造函数
如果类型具有构造函数,可以像函数一样调用它来创建一个该类型的值。
Converts a value to an array.
Note that this function is only intended for conversion of a collection-like
value to an array, not for creation of an array from individual items. Use
the array syntax (1, 2, 3)
(or (1,)
for a single-element array) instead.
#let hi = "Hello 😃"
#array(bytes(hi))
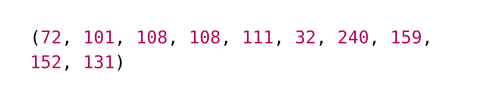
value
The value that should be converted to an array.
定义
函数和类型可以有与其关联的定义 (成员或方法)。可以使用 "." 操作符来访问调用它们。
len
The number of values in the array.
first
Returns the first item in the array. May be used on the left-hand side of an assignment. Fails with an error if the array is empty.
last
Returns the last item in the array. May be used on the left-hand side of an assignment. Fails with an error if the array is empty.
at
Returns the item at the specified index in the array. May be used on the left-hand side of an assignment. Returns the default value if the index is out of bounds or fails with an error if no default value was specified.
index
The index at which to retrieve the item. If negative, indexes from the back.
default
A default value to return if the index is out of bounds.
push
Adds a value to the end of the array.
value
The value to insert at the end of the array.
pop
Removes the last item from the array and returns it. Fails with an error if the array is empty.
insert
Inserts a value into the array at the specified index. Fails with an error if the index is out of bounds.
index
The index at which to insert the item. If negative, indexes from the back.
value
The value to insert into the array.
remove
Removes the value at the specified index from the array and return it.
index
The index at which to remove the item. If negative, indexes from the back.
default
A default value to return if the index is out of bounds.
slice
Extracts a subslice of the array. Fails with an error if the start or index is out of bounds.
start
The start index (inclusive). If negative, indexes from the back.
end
The end index (exclusive). If omitted, the whole slice until the end of the array is extracted. If negative, indexes from the back.
默认:none
count
The number of items to extract. This is equivalent to passing
start + count
as the end
position. Mutually exclusive with end
.
contains
Whether the array contains the specified value.
This method also has dedicated syntax: You can write 2 in (1, 2, 3)
instead of (1, 2, 3).contains(2)
.
value
The value to search for.
find
Searches for an item for which the given function returns true
and
returns the first match or none
if there is no match.
searcher
The function to apply to each item. Must return a boolean.
position
Searches for an item for which the given function returns true
and
returns the index of the first match or none
if there is no match.
searcher
The function to apply to each item. Must return a boolean.
range
Create an array consisting of a sequence of numbers.
If you pass just one positional parameter, it is interpreted as the
end
of the range. If you pass two, they describe the start
and end
of the range.
This function is available both in the array function's scope and globally.
查看示例
#range(5) \
#range(2, 5) \
#range(20, step: 4) \
#range(21, step: 4) \
#range(5, 2, step: -1)
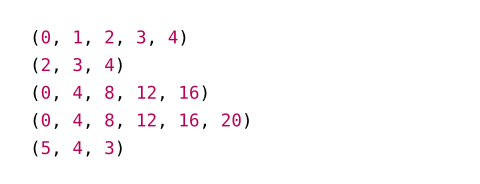
start
The start of the range (inclusive).
默认:0
end
The end of the range (exclusive).
step
The distance between the generated numbers.
默认:1
filter
Produces a new array with only the items from the original one for which the given function returns true.
test
The function to apply to each item. Must return a boolean.
map
Produces a new array in which all items from the original one were transformed with the given function.
mapper
The function to apply to each item.
enumerate
Returns a new array with the values alongside their indices.
The returned array consists of (index, value)
pairs in the form of
length-2 arrays. These can be destructured with
a let binding or for loop.
start
The index returned for the first pair of the returned list.
默认:0
zip
Zips the array with other arrays.
Returns an array of arrays, where the i
th inner array contains all the
i
th elements from each original array.
If the arrays to be zipped have different lengths, they are zipped up to the last element of the shortest array and all remaining elements are ignored.
This function is variadic, meaning that you can zip multiple arrays
together at once: (1, 2).zip(("A", "B"), (10, 20))
yields
((1, "A", 10), (2, "B", 20))
.
others
The arrays to zip with.
fold
Folds all items into a single value using an accumulator function.
init
The initial value to start with.
folder
The folding function. Must have two parameters: One for the accumulated value and one for an item.
sum
Sums all items (works for all types that can be added).
default
What to return if the array is empty. Must be set if the array can be empty.
product
Calculates the product all items (works for all types that can be multiplied).
default
What to return if the array is empty. Must be set if the array can be empty.
any
Whether the given function returns true
for any item in the array.
test
The function to apply to each item. Must return a boolean.
all
Whether the given function returns true
for all items in the array.
test
The function to apply to each item. Must return a boolean.
flatten
Combine all nested arrays into a single flat one.
rev
Return a new array with the same items, but in reverse order.
split
Split the array at occurrences of the specified value.
at
The value to split at.
join
Combine all items in the array into one.
separator
A value to insert between each item of the array.
默认:none
last
An alternative separator between the last two items.
intersperse
Returns an array with a copy of the separator value placed between adjacent elements.
separator
The value that will be placed between each adjacent element.
sorted
Return a sorted version of this array, optionally by a given key function. The sorting algorithm used is stable.
Returns an error if two values could not be compared or if the key function (if given) yields an error.
key
If given, applies this function to the elements in the array to determine the keys to sort by.
dedup
Deduplicates all items in the array.
Returns a new array with all duplicate items removed. Only the first element of each duplicate is kept.
查看示例
#(1, 1, 2, 3, 1).dedup()

key
If given, applies this function to the elements in the array to determine the keys to deduplicate by.